Unlocking Career Opportunities: Key Core Java Coding Interview Questions
When it comes to landing a job in the software development industry, it’s important to have a strong foundation in core Java coding. Whether you’re a fresh graduate or an experienced professional looking to switch careers, being prepared for core Java coding interview questions can give you the edge you need to stand out from the competition. In this article, we will explore some of the most commonly asked core Java coding interview questions and provide valuable insights on how to answer them.
What is the difference between an abstract class and an interface?
One of the fundamental concepts in Java programming is understanding the difference between abstract classes and interfaces. Both serve as blueprints for creating classes, but they have distinct characteristics.
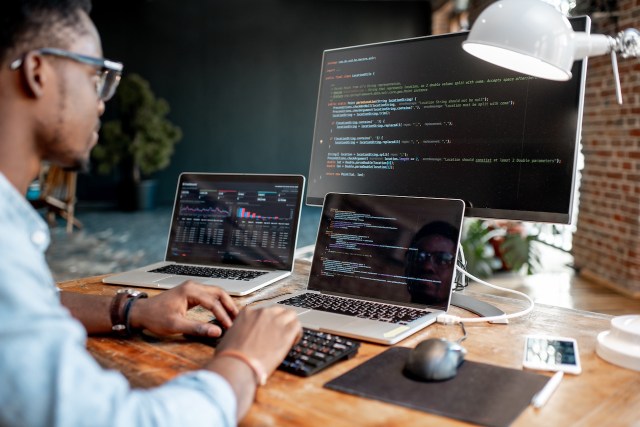
An abstract class is a class that cannot be instantiated and is meant to be subclassed by other classes. It can contain both concrete methods (methods with implementation) and abstract methods (methods without implementation). Subclasses of an abstract class must implement all its abstract methods.
On the other hand, an interface is a collection of method signatures without any implementation. It defines a contract that classes must adhere to by implementing all its methods. Unlike abstract classes, interfaces cannot have any instance variables or concrete methods.
When answering this question in an interview, it’s important to highlight these differences and provide real-world examples where each might be used. Showing your understanding of how these concepts work together will demonstrate your grasp of core Java principles.
Explain the concept of multithreading in Java.
Multithreading is crucial in modern software development as it allows programs to execute multiple tasks concurrently, improving performance and responsiveness. In Java, multithreading is achieved through the use of threads – lightweight processes within a program that can run independently.
To create a thread in Java, you can either extend the `Thread` class or implement the `Runnable` interface. The `Thread` class provides more flexibility, but implementing the `Runnable` interface is often preferred as it allows for better separation of concerns.
When discussing multithreading in a Java coding interview, it’s important to cover concepts such as thread synchronization, deadlock prevention, and thread pooling. Demonstrating an understanding of how to handle concurrent access to shared resources and avoid potential pitfalls will showcase your ability to write efficient and robust multithreaded code.
What are the differences between checked and unchecked exceptions?
Exception handling is a critical aspect of Java programming that helps deal with unexpected scenarios during runtime. In Java, exceptions are classified into two categories: checked exceptions and unchecked exceptions.
Checked exceptions are exceptions that must be explicitly declared in the method signature or caught using a try-catch block. These exceptions typically represent recoverable conditions that occur outside the control of the program, such as file not found or network connection issues. By forcing developers to handle these exceptions explicitly, Java enforces more robust error handling practices.
On the other hand, unchecked exceptions (also known as runtime exceptions) do not require explicit handling or declaration. These exceptions usually indicate programming errors such as null pointer dereference or array index out of bounds. Unchecked exceptions can be avoided by writing defensive code and performing proper input validation.
In an interview setting, it’s important to understand when to use checked and unchecked exceptions appropriately. Discussing best practices for exception handling and demonstrating your ability to handle different types of exceptions will impress potential employers.
How does garbage collection work in Java?
Garbage collection is an automatic memory management feature in Java that frees up memory occupied by objects that are no longer referenced by any part of the program. This process helps prevent memory leaks and eliminates the need for manual memory management.
In Java, garbage collection works by periodically identifying objects that are no longer reachable through references from the root of execution (e.g., main function or active threads) and reclaiming their memory. The Java Virtual Machine (JVM) has a built-in garbage collector that handles this process.
When discussing garbage collection in an interview, it’s important to understand how the JVM manages memory, different types of garbage collectors (such as the mark-and-sweep algorithm), and techniques for optimizing garbage collection performance. Showing your knowledge of memory management in Java will demonstrate your ability to write efficient and scalable code.
In conclusion, preparing for core Java coding interview questions is essential for anyone looking to excel in the software development industry. By understanding concepts such as abstract classes and interfaces, multithreading, exception handling, and garbage collection, you can showcase your expertise and increase your chances of landing that dream job. Remember to practice answering these questions beforehand and provide clear and concise explanations during the interview. Good luck.
This text was generated using a large language model, and select text has been reviewed and moderated for purposes such as readability.